10 Common Data Structures Every Programmer Must Know
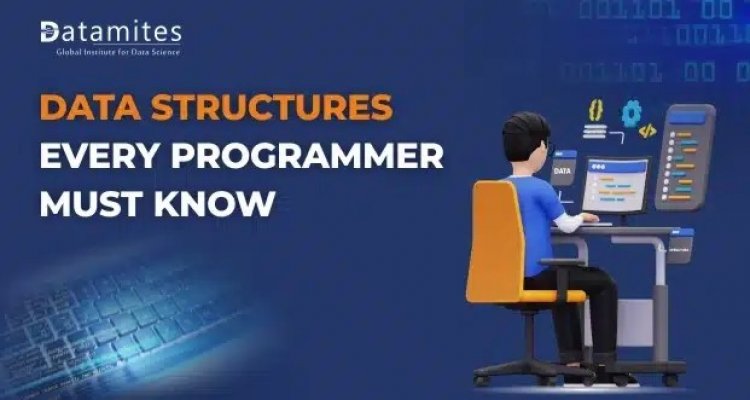
Data is vital and it is key to everything in this digital era. For any business, whether large-scale or small-scale, data becomes an essential tool for the business activity, for instance, expanding the business or finding a solution for any specific problem in the business or making a particular decision, for everything data is required.
Each and every decision that we make today is a data-driven decision. We deal with massive amounts of data and hence it becomes important to store the data and make it easily accessible. When the data is organised properly, it reduces the time complexity and makes it easy for us to perform any critical operations on the data. But how do keep it organised? This is where the concept of Data Structures came into existence.
So, what is data structure?
To tell in simple terms, it is a way of storing and organizing the data in the computer which certainly helps in easy retrieval of data and using the data more efficiently. It is not a programming language, but it is used by all programming languages. It is a set of algorithms used to structure the data in computer memory. The data that we collect, could be numerical or categorical type. So, there are specific data structures, which store the data of the same data type. You can imagine the easiness of accessing or performing any operation when the data belongs to the same data type.
In this article, we will discuss the importance of data structure and the common data structures that everyone should know about.
Before going into the topic, let’s understand the difference between data type and data structure;
To make it simple Data type is the classification of data which tells what is the form of the data/information we have. It basically tells the type of any variable used in our code. For example, whether the data is of integer type or string type or of Boolean type and also tells how much memory space is required to store it. Whereas Data Structures help us to keep our data organized in the memory for easy access or for performing any operations like searching, inserting, deleting, sorting etc or for any modifications in the data.
Refer to the article: Support Vector Machine Algorithm (SVM) – Understanding Kernel Trick
Importance of Data Structure:
For any programming language, data structures are important which sets the rule for storing the data. It arranges the information such that if any specific data is required that can be searched quickly with the help of methods instead of going through every record. Data Structures are not only used for storing or organizing the data but also for processing and for retrieving the data. For instance, when we wanted to fetch 1 record from millions of records in the database, without data structure it becomes a tedious job. Data structures provide various methods for all the operations to perform in a database easily.
The basic types of data structures are Primitive and Abstract Data Structures. Primitive is the fundamental data structures like integer, Boolean, string, float etc. Abstracts are complex data structures like arrays, linked lists, queues, trees, etc that can handle a large amount of data.
Let us discuss briefly the different data structures that are commonly used. To be more specific first let us see the data structures in Python along with other common data structures.
Read this article: A Complete Guide to Stochastic Gradient Descent (SGD)
Types of Data Structures:
There are many Data Structures that are used in almost every programming language. The data structures are classified broadly based on the arrangement of the elements in the memory. Some are arranged in a sequence which is called a linear data structure and some are not in a sequence manner which is referred to as a non-linear data structure. Here let’s see what are the types of Data Structures;
1. Array:
The array is a very common and basic data structure. In many programming languages, we have arrays like Python, C etc. Arrays are the collection of data items that are stored in a contiguous memory location. The array can be used for storing elements of different data types like integers, float, etc. But each array can store only the homogeneous elements ie.,
elements of the same data type.
It is also called a static linear data structure since the size and the memory location is fixed during the compilation time. Hence, we need to know the maximum required size to store the elements. The elements in the array have an index which gives the position of the elements. It is unique and it is used to identify or access the elements. The index always starts with zero, hence the first element will have an index of zero, the second element will have an index of 1 and so on. The concept of contiguous memory location prevents the wastage of memory. But this is also a disadvantage. Since the size is fixed, you cannot store extra information if required.
Also refer to this article: A Complete Guide to Linear Regression Algorithm in Python
2. Linked List:
Linked List is the collection of data elements but unlike Arrays, they aren’t stored in contiguous memory location. They are ordered not by the physical memory location but by the logical links which are included in the element itself. The linked list has nodes or elements which contain two things, the data field and the pointer to connect to the next node as shown below:
The starting node is the head node and the last node will be pointing to None which tells that it is the end of the list.
It creates a link between the data elements. This helps in efficient memory utilization. Unlike Arrays, it can allocate the memory if we want to add new elements. Also, it can deallocate the memory if not required. Hence size is not fixed. But it requires more memory as it has to include a pointer in each element.
Swap first and last element in list
3. Tuple
A tuple is a linear data structure that stores the element in a sequence. It is indexed hence the elements can be accessed the elements using an index or slice operation.
4. Dictionary
Dictionary is also used to store a collection of data where the elements will be as a key-value pair. Here each value is mapped to a unique key value. So, to add or remove any elements, or to do any modifications, we use keys to carry out those operations.
5. Queue:
It is a linear data structure, where the elements are stored in a sequential order such that the first element only can be removed first like FIFO (First In First Out) method. It is just like a normal queue where the customer who comes in first will go out first. Similarly, you can insert elements on one end and delete/remove elements at the other end as shown below;
Adding the new element to the queue is called Enqueue and removing the element from the queue is called Dequeue.
6. Stack:
It also stores the element in sequential order. The only difference between Stack and Queue is that Stack follows the LIFO (Last In First Out) method, where the element that was inserted last will be removed first as shown below;
7. Sets:
In this data structure, the elements are not stored in any particular order. It does not take duplicate elements. We can also perform set operations like Union, Intersection, Difference, etc between two or more sets. Let’s say when the following operations happen on the 2 sets A and B
- Union: A new set is created by combining all the elements of sets A and B.
- Intersection: A new set is created with the common elements of sets A and B.
- Difference: It gives a new set with the elements that exist only in one set.
Convert a list into set using Set function
8. Graphs:
The graph is a non-linear data structure since it does not store elements in a sequence. It is a pictorial representation of objects which looks like a network. Here the objects are interconnected through edges. Graphs basically are a set of vertices and edges where vertices are the nodes that contains information like Name, Age etc and edges are the lines that connect the nodes.
9. Trees:
This data structure is also a non-linear data structure. It follows a hierarchical data structure (tree-like structure) which consists of nodes called root nodes, internal nodes and leaf nodes. Each node is an entity which contains information or some values in it. The root node is the topmost node and only one node will be a root node. The internal nodes are split and have at least one child node. Leaf nodes are the terminal nodes where further split doesn’t happen. These nodes are connected by links called edges. This structure helps in quick and easy access to the data.
The difference between Tree and Graph is that tree is represented in aa hierarchical (tree-like) structure whereas the latter represents data like a network. Graphs don’t have root nodes but the Trees have.
10. Hash Table:
Like dictionary, this data structure also stores the data as a key value pair and maps the keys to the values. But unlike dictionary, in hash table, the elements can be of any data type for keys and the values. The elements are not ordered the way they are inserted. Here, multiple elements can have same index. This is called as hash collision. To reduce the hash collision, chaining is used where it stores the elements in the same index by using a doubly-linked list. When more elements are given with the same index, then a list is created to store all those elements.
Conclusion:
Data structures makes the program very efficient by reducing the time complexity. Also helps in efficient storage of large data. With a proper data structure, we can easily manipulate large amount of data. Well organised data structures can help us save a lot of processing time while doing any operations like deletion, insertion, retrieval etc. We can use them directly even with a minimal amount of technical knowledge.
Being a prominent data science institute, DataMites provides specialized training in topics including machine learning, deep learning, Python course, the internet of things. Our artificial intelligence at DataMites have been authorized by the International Association for Business Analytics Certification (IABAC), a body with a strong reputation and high appreciation in the analytics field.
Set an Index of Dataset