Getting Started with Python: A Beginner’s Guide
"Getting Started with Python: A Beginner’s Guide" offers a simple introduction to Python programming, covering the basics and helping newcomers start coding confidently. Perfect for those eager to learn Python from scratch!
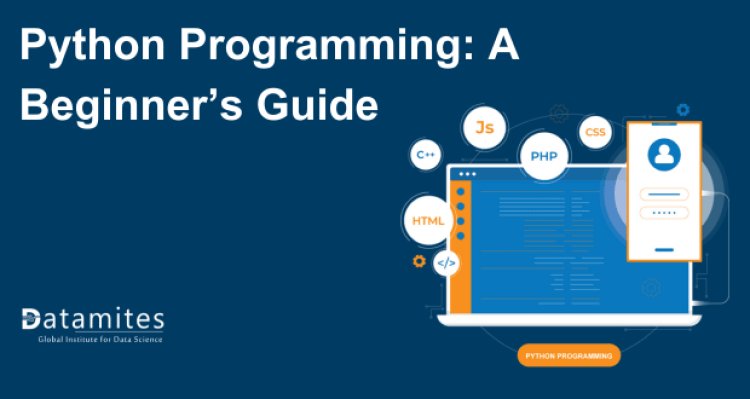
Python is a computer language that has gained immense popularity due to its versatility and capability. Whether you're interested in data science, web development, or automation, Python is a fantastic starting point. This guide is designed to introduce you to Python from the ground up, offering clear explanations and practical steps to get you started.
Introduction to Python: A Gateway to Modern Programming
High-level interpreted Python is renowned for emphasizing readability and simplicity of usage. Developed by Guido van Rossum and introduced in 1991, Python has since become one of the most widely used programming languages globally. Its straightforward syntax and versatility make it a great option for both novice and seasoned programmers.
The global Python market is projected to reach USD 100.6 million in 2023, with a revenue CAGR of 44.8% from 2022 to 2030. Key drivers include increasing use in web and software development, rapid adoption of Industry 4.0 technologies, growing reliance on data analytics, preference over other programming languages, and rising demand for real-time IoT and edge computing solutions.
The Importance of Learning Python
Learning Python opens doors to numerous career opportunities in various fields, from web development to artificial intelligence. Its user-friendly nature makes it easier to grasp fundamental programming concepts, which can be transferable to other languages.
What is Python Programming?
Python was created with the goal of making programming more accessible and efficient. Unlike other languages that focus heavily on syntax, Python prioritizes code readability. Its design philosophy focuses on simplicity and elegance, which makes it a popular choice among developers.
Key Features and Benefits of Python Programming
Python’s key features include:
Readability: Clear and understandable syntax.
Versatility: Ideal for web development, data analysis, AI, and beyond.
Community Support: A vast and active community offering numerous resources.
Extensive Libraries: A robust library and framework ecosystem supporting a large number of applications.
Setting Up Python
Downloading and Installing Python
To start coding in Python, you'll need to install it on your machine. Here is a detailed tutorial for several operating systems:
Windows
- Visit the Python official website.
- Download the latest version for Windows.
- Make sure you choose the option to add Python to your PATH when you run the installer.
macOS
- Go to the Python official website.
- Download the macOS installer.
- After downloading the file, open it and adhere to the setup guidelines.
Linux
- Open your terminal.
- Use your package manager to install Python, e.g., sudo apt-get install python3 for Debian-based distributions.
Introduction to Python IDEs (Integrated Development Environments)
An IDE boosts coding productivity by providing tools and features like debugging capabilities and code recommendations. Here are a few popular ones:
- PyCharm: A powerful IDE with extensive features for professional development.
- VS Code: A lightweight editor that offers a broad selection of extensions.
- Jupyter Notebook: Perfect for interactive coding and data analysis.
Read these articles:
- Why Python is Essential for Data Science and AI
- Comprehensive Guide for Python and R Programming
- Guide to Python Programming Career
Python Basics: Building a Strong Foundation
Building a strong foundation in Python involves understanding its basic concepts and syntax. Here’s a distinctive approach to help you begin:
Variables and Data Types
- Variables: Named containers for storing data.
- Data Types: The type of data stored in variables. Key types include:
- Integers: Whole numbers.
- Floats: Decimal numbers.
- Strings: Sequences of characters.
- Booleans: True or False values.
Operators
- Arithmetic Operators: Used for mathematical operations (e.g., addition, subtraction).
- Comparison Operators: Used to compare values, such as checking for equality or inequality.
- Logical Operators: Used to combine or invert conditions (e.g., and, or, not).
Input and Output
- Input: Getting data from the user.
- Output: Displaying data to the user.
Control Flow
- Conditional Statements: Allow you to execute certain blocks of code based on conditions (e.g., making decisions).
- Loops:
- For Loops: Used for iterating over sequences (like lists or strings).
- While Loops: As long as a particular condition holds true, continue executing the code.
Grasping these fundamentals will provide you with a strong base for tackling more advanced Python programming concepts.
Core Python Concepts: Diving Deeper
Diving deeper into core Python concepts involves exploring advanced features and idioms that can help you write more efficient, readable, and Pythonic code. The following are some places to think about:
Data Structures
Python provides several built-in data structures to handle data effectively:
Lists:
- Description: Ordered collections that can be modified.
- Characteristics: They can contain elements of different types and support various operations such as appending, removing, and accessing elements by index.
Tuples:
- Description: Immutable ordered collections.
- Characteristics: Once created, the elements cannot be changed. Tuples are commonly employed to bundle related information together.
Dictionaries:
- Description: Collections of key-value pairs for quick lookups.
- Characteristics: Keys must be unique and immutable, while values can be of any type. Dictionaries are ideal for associative arrays or mappings.
Sets:
- Description: Unordered collections of unique elements.
- Characteristics: Beneficial for removing duplicate entries and testing membership. Sets do not support indexing, slicing, or other sequence-like behaviors.
Object-Oriented Programming (OOP)
OOP is a programming methodology that models and represents real-world entities using objects and classes:
Classes and Objects:
- Description: Classes are blueprints for creating objects. Objects are specific instances of classes that contain attributes (data) and methods (functions).
- Characteristics: Classes define the structure and behavior of objects, while objects represent specific instances with their own state.
Inheritance:
- Description: Allows new classes to inherit attributes and methods from existing ones.
- Characteristics: Facilitates code reuse and establishes a hierarchical relationship between classes. A derived class can extend or override the behavior of its base class.
Polymorphism:
- Description: The ability to use a unified interface for different data types.
- Characteristics: Enables objects of different classes to be treated as instances of the same class through a common interface, often achieved through method overriding and overloading.
Encapsulation and Abstraction:
- Description: Encapsulation means concealing the internal workings and only revealing the essential features. Abstraction focuses on exposing the essential features of an object while hiding complex implementation details.
- Characteristics: Encapsulation is achieved through access modifiers and properties, while abstraction is achieved through abstract classes and methods.
Modules and Packages
Modules and packages help in organizing code efficiently:
Importing Modules:
- Description: Use the import statement to bring in standard or custom modules.
- Characteristics: Modules are files containing Python code, and importing them allows access to their functions, classes, and variables.
Creating Modules and Packages:
- Description: Structure your code into reusable components by creating modules and packages.
- Characteristics: A package is an assemblage of modules arranged in a directory structure, whereas a module is a single file. Packages facilitate modular design and code reuse.
By understanding and effectively using these core concepts, you can write more efficient and organized Python code.
Read these articles:
- What is Certified Python Developer?
- What is Datamites Certified Data Analyst Certification
- What is Certified Data Scientist Course
Advanced Python Topics: Expanding Your Knowledge
Expanding your Python knowledge to more advanced topics can greatly enhance your programming skills and open up new possibilities. Here are some advanced Python topics you might explore:
Error and Exception Handling
To ensure your code runs smoothly, handling errors effectively is crucial. Key aspects include:
- Types of Errors: Understand different errors such as syntax errors (mistakes in code structure), runtime errors (problems that occur during execution), and logical errors (flaws in the program's logic).
- Try-Except Blocks: Use the try block to execute code that might cause errors, and the except block to handle those errors gracefully.
- Custom Exceptions: Create your own exception classes to manage specific error conditions in your code.
File Handling
Python allows for various operations on files:
- Reading Files: Open files for reading using appropriate modes, such as 'r' for text and 'rb' for binary.
- Writing and Appending: Modify files by opening them in write ('w') or append ('a') modes.
- Different File Formats: Handle a variety of file formats, such as CSVs, JSON, and text files.
Regular Expressions
Regular expressions (regex) help with text pattern matching:
- Pattern Matching: Utilize regex to find and manipulate text based on specific patterns.
- Text Manipulation: Use regex to perform operations like searching, replacing, and splitting strings.
Functional Programming
Python supports functional programming techniques:
- Higher-Order Functions: Functions that take in parameters from other functions.
- Lambda Expressions: Create anonymous, short-term functions for quick use.
- Map, Filter, Reduce: Apply these functions to process collections of data effectively.
Decorators
Enhance the functionality of your functions with decorators:
- Function Enhancement: Use decorators to modify or extend the behavior of functions without altering their core code.
Python Libraries and Frameworks: Tools for Success
Python is an effective language for many different kinds of applications because of its robust ecosystem of libraries and frameworks. There's probably a technology out there that works for you, whether you're working on automation, machine learning, data analysis, or web development. Here's an overview of some of the most popular Python libraries and frameworks:
- NumPy: Offers support for large, multi-dimensional arrays and matrices, as well as a range of mathematical functions to manipulate these arrays. It is a fundamental component of Python scientific computing.
- Pandas: Supplies the functions and data structures needed to work with structured data effectively. It's frequently used for analysis and data manipulation, particularly with data frames.
- Matplotlib: A plotting library that produces figures and plots in various formats and interactive environments. It's essential for visualizing data and creating static, animated, and interactive plots.
- Scikit-learn: Simple and effective tools for data mining and analysis are included in this machine learning package. With support for a large variety of machine learning methods, it is based on NumPy, SciPy, and Matplotlib.
- Requests: A straightforward HTTP library designed for easily sending requests to web servers. It simplifies tasks like fetching data from web pages or interacting with RESTful APIs.
- Beautiful Soup: A tool for interpreting and analyzing HTML and XML files. It's very helpful for web scraping, which lets you take information off of websites.
- Django: A top-tier web framework that promotes swift development and a sleek, practical design. Numerous features are pre-installed, such as an admin interface and an ORM.
- Flask: A more versatile, lighter, and simpler web framework than Django. It allows developers to select the libraries and tools that best suit their needs.
Each of these tools has its strengths and is suited to different tasks, making Python a versatile language for a variety of applications.
Python for Specific Domains: Applying Your Skills
Python's versatility makes it ideal for specialized domains, allowing professionals to tailor its capabilities to their specific needs. A brief summary of using Python expertise in particular domains is provided below:
- Data Science: For tasks like data manipulation, analysis, and visualization, Python is essential in data science. Libraries such as Pandas, NumPy, and Matplotlib facilitate data handling and plotting, while SciPy and StatsModels support complex statistical analysis.
- Web Development: Python's frameworks like Django and Flask streamline web development by providing tools and libraries to create robust web applications. Django offers a high-level structure with built-in features, while Flask provides a more flexible and lightweight option.
- Machine Learning: For machine learning, Python is the preferred language. Libraries such as TensorFlow, Keras, and scikit-learn enable the development of sophisticated models for tasks like classification, regression, and clustering.
- Automation/Scripting: Python is excellent for automating repetitive tasks. With libraries like Selenium for web scraping and automation, and scripts for file handling and system operations, Python simplifies many routine processes.
- Finance: In finance, Python is used for quantitative analysis, algorithmic trading, and financial modeling. Libraries like QuantLib and pandas-datareader assist in handling financial data and executing trading strategies.
- Game Development: Python’s Pygame library supports game development by providing tools for graphics, sound, and game physics, making it a good choice for prototyping and simple game projects.
Refer these articles:
- Python Programmer Career Scope
- What would be the Python Course Fee in India?
- Python Course Fee in Bangalore
Python Projects and Challenges
Python challenges and projects are great ways to improve your programming abilities and get practical experience with real-world applications.
Python Projects
Here are some engaging Python project ideas that can help enhance your programming skills:
- Web Scraping Tool: Develop a script to extract data from websites using libraries like Beautiful Soup and Scrapy.
- Automated Email Sender: Create a program to send emails automatically using the smtplib library.
- To-Do List App: Build a command-line or GUI-based to-do list application using Tkinter.
- Weather App: Create a weather forecasting application that fetches data from an API like OpenWeatherMap.
- Personal Finance Tracker: Develop a program to track and visualize personal expenses and savings.
- Chatbot: Design a simple chatbot using NLP libraries like NLTK or spaCy.
Global Python Programmer Salaries
Python programming remains a pivotal force in the global tech industry, significantly impacting salary trends for professionals across the world. As the demand for Python expertise continues to rise across diverse sectors, it's crucial to understand how these trends affect programmer salaries. Overall, Python programmers are enjoying competitive compensation worldwide, underscoring the strong demand for their skills in the job market. Here's the Python programmer salary trend across different regions:
- In the United States, Python Developers are in high demand across various sectors such as technology, finance, and healthcare. On average, they earn around $1,18,631 per year, according to Glassdoor.
- In the United Kingdom, particularly in London, a prominent global financial and tech hub, the average annual salary for a Python developer is approximately £80,482, as reported by Indeed.
- In India, with its rapidly growing tech industry and key hubs in Bangalore, Hyderabad, and Pune, the average salary for a Python Developer stands at ₹5,77,500 per year, according to Glassdoor.
- South Africa's tech industry, centered in cities like Cape Town and Johannesburg, offers Python Developers an average salary of about R 641,654 per year, according to Indeed.
- In Singapore, a major business and tech center in Asia, Python Developers earn an average salary of $104,452 per year, as noted by Indeed.
Key Python Challenges
Python is a versatile and widely-used programming language, but it presents several challenges that developers often encounter. Here are some key challenges:
Algorithm Optimization:
- Improve the effectiveness and performance of current algorithms.
- Focus on reducing time complexity and improving resource utilization.
Code Refactoring:
- Revise the code to improve its clarity and ease of maintenance.
- Apply best practices and design patterns.
Unit Testing:
- Write comprehensive unit tests for a given codebase.
- Utilize testing frameworks such as unittest, pytest, or nose.
Concurrency Handling:
- Solve problems involving concurrency and parallelism.
- Implement multithreading and multiprocessing to handle tasks efficiently.
Error Handling:
- Boost a project's error handling and debugging.
- Implement robust exception handling and logging mechanisms.
Embarking on your Python journey opens a world of possibilities, from developing software to exploring data and creating intelligent systems. As you progress from basic syntax to advanced programming techniques, you'll gain the skills needed to tackle real-world challenges and innovate in various fields. Python's versatility and ease of learning make it an ideal choice for both beginners and seasoned developers alike. By mastering Python, you're not just learning a programming language, you're equipping yourself with a powerful tool for your future career.
To further enhance your Python skills, consider enrolling in DataMites Institute Certified Python Developer course. DataMites offers globally recognized certification that can significantly boost your career prospects. Additionally, DataMites provides specialized courses in Python for Data Science, Python for Artificial Intelligence, Python for Deep Learning, and Python for Machine Learning. With these targeted programs, you can deepen your expertise and stay ahead in the rapidly evolving tech landscape.
DataMites offers advanced Python programming training, equipping you with the skills needed for real-world software development and data analysis. The course covers essential Python concepts, practical projects, and real-world applications. Upon completion, you'll receive certification from IABAC and NASSCOM FutureSkills. With over ten years of experience, DataMites has empowered more than 100,000 learners globally, making it a leader in Python education.