Append Function in Python
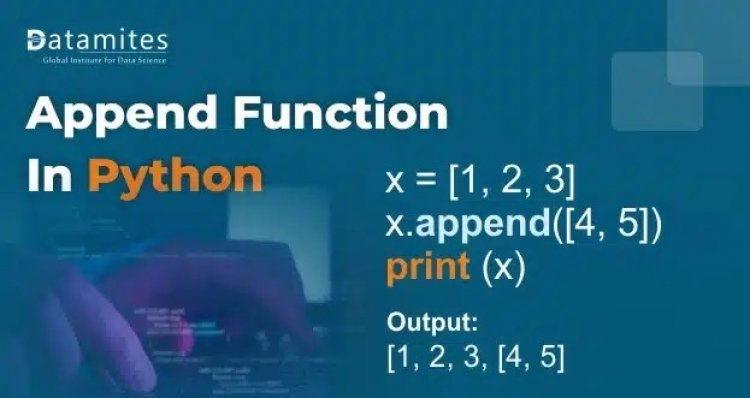
Origin:
Python is a comprehensive programming language with many tools and packages. It makes sense why it is the most extensively used programming language in the world among developers. Its English-like grammar and the wide range of features that come with it are what make it a flexible language. The Python append function, which we’ll discuss today, is one of those operations. You will become familiar with the append function’s definition, significance, and distinctive features. There are a set number of built-in data structures in every programming language. In essence, data structures are systems that keep the data organized so that we may make use of it.
The significance of Python’s append function in List:
When working with lists in Python, the append function is extremely useful. Lists are similar to arrays in that they don’t always need to store data of the same data type. As a result, lists are among Python’s most popular and robust tools. The fundamental distinction between lists and arrays is that the former can use multiple data types. Lists are mutable. In other words, you can make modifications to a list even after it has been generated. Let’s look at how to create, modify, delete, and append an object in a list.
Read this article: Support Vector Machine Algorithm (SVM) – Understanding Kernel Trick
Creating a list
my_list = [“Apple”,”Banana”,”Cucumber”] print(my_list)
out: [‘Apple’, ‘Banana’, ‘Cucumber’]
Appending a number
my_list.append(1)
print(my_list)
out: [‘Apple’, ‘Banana’, ‘Cucumber’, 1]
Appending a string
my_list.append(‘Pineapple’)
print(my_list)
Out: [‘Apple’, ‘Banana’, ‘Cucumber’, 1, ‘Pineapple’]
Appending a float
my_list.append(2.4)
print(my_list)
out:[‘Apple’, ‘Banana’, ‘Cucumber’, 1, ‘Pineapple’, 2.4]
Appending a list
my_second_list = [1,2,3,4,5]
my_list.append(my_second_list)
print(my_list)
out: [‘Apple’, ‘Banana’, ‘Cucumber’, 1, ‘Pineapple’, 2.4, [1, 2, 3, 4, 5]]
Refer to the article: A Complete Guide to Stochastic Gradient Descent (SGD)
Python’s extend() Function:
Python‘s append() function adds a single item to the end of the provided list by accepting it as an input parameter. In truth, Python’s append() function returns nothing at all rather than a new list of things. Simply by including the item at the end of the list, the original list is modified. So, after appending, the size of the original list gets increased by 1. A list has the ability to store multiple data types of objects including strings, integers, float, Boolean, and in fact a list as well. When a list itself is appended to a list, then it behaves as a single object. What if, however, we wished to include every entry from one list in another list? Yes, that is doable and may be accomplished with ease using the extend() function. Understanding the distinction between the extend function and the append function can be challenging for Python newbies. Both of these increase the length of a list, but the primary distinction is how many elements they add to the list at once. One item is added to the end of a list using the append function. The extend function, on the other hand, loops over the input and adds each item from its list to the particular list. The index of a list rises by one when using the append function, but by the number of elements in the extended list when using the extend function.
Let us look at how extend() function is used:
my_frst_lst = [‘hello’,’people’] my_scnd_lst = [1,2,3,4] my_frst_lst.extend(my_scnd_lst) print(my_frst_lst)
out: [‘hello’, ‘people’, 1, 2, 3, 4]
Also read this article: A Complete Guide to Linear Regression Algorithm in Python
Most Popular append() function interview question:
Q1) How do Python’s insert() and append() functions vary from one another?
With Python’s insert() function, you can add a list element at any index you choose. In contrast, append() merely adds the entry to the list’s end.
Q2) How do we append to a list in Python?
You can employ itertools.chain(), extend(), and append().
Q3) What are the input and output parameters for Python’s append() function?
The item to append is the only parameter required by the append() method in Python. As it alters the original list by adding the item at the end of the list, it does not return any value or list.
Q4) Does Python’s append() method create a copy of the list?
Not at all. append() function changes the initial list. The list is not copied in any way.
Q5) How does Python’s extend() and append() functions differ from one another?
Unlike Python’s append() function, which adds a single element to the list’s end, extend() loops through the elements in its parameter, adding each one to the list before extending it.
Being a prominent data science institute, DataMites provides specialized training in topics including machine learning, deep learning, Python course, the internet of things. Our artificial intelligence at DataMites have been authorized by the International Association for Business Analytics Certification (IABAC), a body with a strong reputation and high appreciation in the analytics field.
Append List Method in Python
Extend List Method in Python
Create Nested list using function