Python Tuples and When to Use them Over Lists
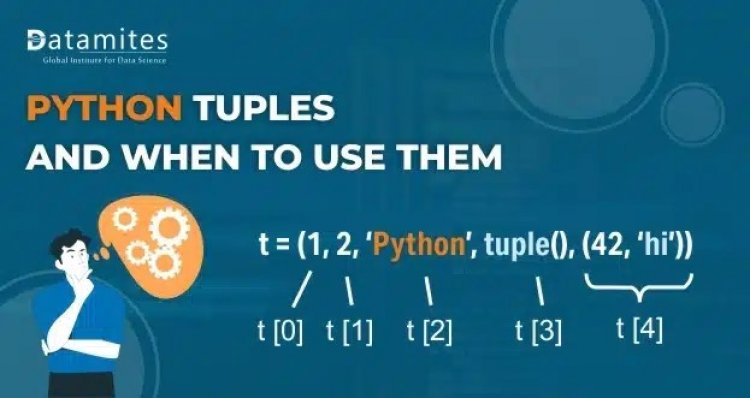
The python language can be looked upon as an all-encompassing platform that speaks to its user in plain words. Data analysts or programmers may have a need to perform various operations upon their data, often in ways to allow for flexibility, but also at times to preserve the inherent nature of the data. The predefined data structures within Python work very well to satisfy such requirements.
Types of Data Structures:
In a nutshell, a data structure can be defined as a means to present collections of a certain number of objects which may or may not be in a specific sequence. A typical operation to be performed within Python will almost certainly require the assistance of a data structure. Depending on the scenario, the different types of structures come in handy for tackling varied types of data.
The four prominent ones we utilize are lists, tuples, sets, and dictionaries. An often posed question is when to use tuples and how different they are from lists. Here we will look to address the very question.
Refer this article: A Complete Guide to Stochastic Gradient Descent (SGD)
What is a List?
Lists – A list is a collection of data objects wherein the order is stated beforehand. The key benefit of making the order or sequence apparent is to be able to call certain elements with the help of indexing. Therefore the words ‘ordered’ and ‘indexed’ are used commonly when dealing with lists. Let us take a look at examples of lists.
list1= [23,45,6,32,81,90]
list2= [5.9, 7.6, 4.4, 3.4, 3.6]
list3= [‘words’, ’data_scientist’, ‘AI’, ‘machines’]
‘list1’ above is a simple collection of integers, where each element except the last is separated from the others with a comma. You may notice the elements of the list are enclosed in square brackets, which is the standard indicator for a user that we are working with a list.
Likewise, a list of pure float values as in ‘list2’, or purely strings may also be created. While there is no restriction on creating a list with mixed data types, such a list is less prevalent in usage.
Read this article: A Complete Guide to Linear Regression Algorithm in Python
What is a Tuple?
Tuples are ordered collections of elements which are immutable in nature; that is to say, we cannot add, remove or replace the elements of a tuple previously defined. We may be able to create a new tuple or delete an existing one, but never make changes to them.
To be clear, this is quite opposite in nature to how a list operates – there is no flexibility to a tuple. Once assigned, the elements cannot be modified howsoever.
tup1 = (23,65,47,83,22,44) # a tuple of integers
Tuples are identified with regular parentheses. We can create tuples of different data types and they permit mixed object types as well.
Also read this article: What is a Confusion Matrix in Machine Learning?
Choosing a Tuple over a List:
If it must come to a grouping of data where the original input must be preserved at all costs, we are basically calling for the immutability of a tuple most required.
The example we now consider is for monitoring the impact of exercising under a prescribed routine.
Convert a list to string with Join Function in Python
Use Case to Compare the Two:
Given a dataset for people from within a group of gym-goers, let us assign the data to various lists. Each list pertains to different parameters about the group.
Gymlist_names= [‘Mac’, ‘Om’, ‘Sarah’, ‘Trisha’, ‘Amar’, ‘Chandra’, ‘Tom’, ‘Ira’, ‘Vinod’, ‘Esha’]
Gymlist_weights= [64, 67, 55, 51, 58, 80, 69, 48, 72, 61]
Gymlist_BMI= [22, 23, 25, 20, 19, 28, 21, 22, 20, 19]
There are three lists in all with the data containing the names of people under study, their weights in kg, and the body mass index of each participant. To be sure we’d probably have a dozen parameters more but let’s keep it simple and stick to these.
Assuming the data about the gym-goers is fairly recent, we may look at adding or removing certain participants from the study. We may also want to replace a few of them based on future availability for the study. For all these operations, our lists can easily be modified; the built-in functions of python would deliver for us.
Now consider a study of a certain performance enhancement on this very group of people. Let us assume the group shows consistent results which prompt the drug company to hold them as a reference group for all future studies (for which of course, they offer incentives and the gym-goers willingly comply).
If the firm makes no distinction between the members of the group, they retain and fix the sequence to match the original list. We now create a tuple with the same retained order.
GymNames_tup= (‘Mac’, ‘Om’, ‘Sarah’, ‘Trisha’, ‘Amar’, ‘Chandra’, ‘Tom’, ‘Ira’, ‘Vinod’, ‘Esha’)
GymWts_tup= (64, 67, 55, 51, 58, 80, 69, 48, 72, 61)
GymBMI_tup= (22, 23, 25, 20, 19, 28, 21, 22, 20, 19)
For the study, the firm assigns a one-week period of observation to the first member, a two-week to the second member, and so on such that the last member of the group is under observation for 10 weeks.
Let us now understand the context of this data in terms of its time value. All changes observed in the parameters will be time-bound. From the firm’s perspective, the order of the members becomes critical so that any deductions and conclusions about the performance of the drug become relatable.
Here is where the tuple’s immutability assumes relevance. We need to ensure that the order and thereby the index of each member and corresponding parameters in the group should not be changeable. We can only rule out such accidents by assigning the entire data to tuples.
Extraction using Partition string method
Python’s Own Usage:
We need not look any further than arrays in Python’s core library of NumPy. Perhaps a clearer case cannot be made for tuples.
Let us import numpy into a jupyter notebook and create an array using the library.
import numpy as np # call the library as np
new_array= np.array([[1,2,3],[4,5,6],[7,8,9]]) # define a two-dimensional array
Once defined, we can call basic attributes of this array such as size to give us the number of elements and ndim to show the number of dimensions.
But let us focus on the shape of the array.
new_array.shape # calling the shape
Out[]: (3, 3)
Notice how the shape is structured by the kernel as a Tuple! We are dealing with an array which happens to be a superior form of data structure in terms of storing multiple elements. However when it comes to expressing the shape, we require the elements within it to be secured, or in other words, the shape must be immutable. Therefore a tuple is the best way to store such data.
Conclusion:
While we state differences between lists and tuples, let us acknowledge the similarities in terms of calling an object by indexing and slicing or allowing for duplicate items within the data structure. We must also bear in mind that advanced functions for data science in python call for the use of a faster data structure. Arrays provide good functional value in this regard over lists or tuples. However, the key advantage of using a tuple rather than any other data structure remains the fact that a tuple has the greatest degree of rigidity.
Being a prominent data science institute, DataMites provides specialized training in topics including machine learning, deep learning, artificial intelligence, the internet of things, data analytics and python Courses. DataMites have been authorized by the International Association for Business Analytics Certification (IABAC), a body with a strong reputation and high appreciation in the analytics field.
Reverse a number in Python